How to Learn Java Programming Language: A Comprehensive Guide
Are you interested in diving into the world of programming? Specifically, do you have a keen interest in learning the widely-used and versatile Java programming language? Look no further! In this comprehensive guide, we will walk you through the process of learning Java programming language from scratch. Whether you are a complete beginner or have some prior coding experience, this article will equip you with the knowledge and resources needed to master Java. So, let's get started!
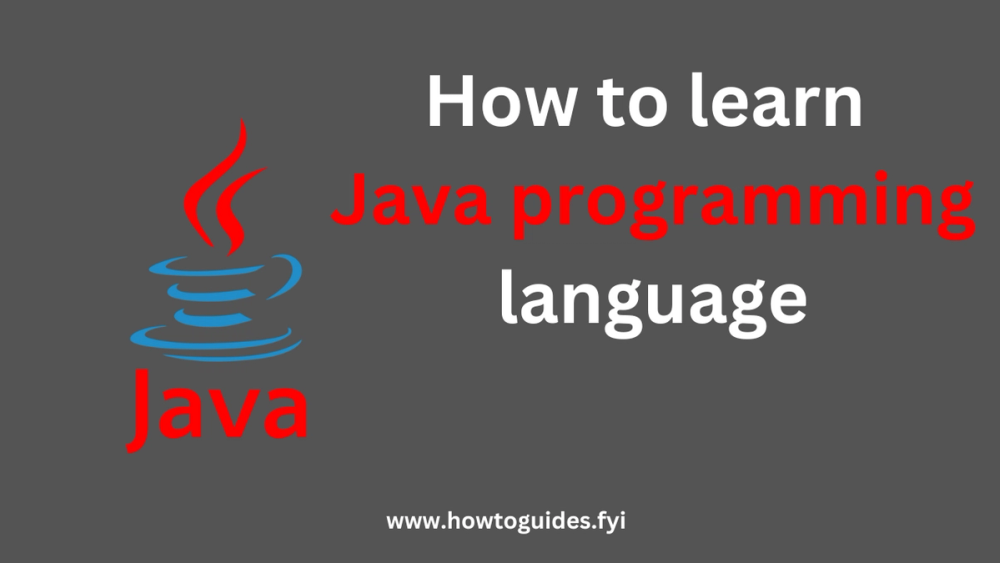
Introduction
Are you interested in diving into the world of programming? Specifically, do you have a keen interest in learning the widely-used and versatile Java programming language? Look no further! In this comprehensive guide, we will walk you through the process of learning Java programming language from scratch. Whether you are a complete beginner or have some prior coding experience, this article will equip you with the knowledge and resources needed to master Java. So, let's get started!
Why Learn Java?
Java is one of the most widely used and versatile programming languages in the world. Here are a few reasons why learning Java is a great choice:
- Platform Independence: Java programs can run on any operating system or platform that supports the Java Virtual Machine (JVM). This means you can develop applications that work seamlessly across different devices and operating systems.
- Large Community and Resources: Java has a vast community of developers who actively contribute to its ecosystem. This means you will have access to a wealth of resources, libraries, and frameworks that can help you solve problems and build robust applications.
- Versatility: Java is used in various domains, including web development, mobile app development (Android), enterprise software development, scientific computing, and more. Learning Java opens up a wide range of career opportunities.
- Object-Oriented Programming: Java follows the object-oriented programming (OOP) paradigm, which is widely recognized as a powerful and scalable approach to building complex software systems. By learning Java, you will gain a solid foundation in OOP concepts.
Setting Up Your Development Environment
Before you start coding in Java, you need to set up your development environment. Here's a step-by-step guide to getting started:
- Install Java Development Kit (JDK): Visit the official Oracle website and download the JDK for your operating system. Follow the installation instructions provided by Oracle.
- Choose an Integrated Development Environment (IDE): An IDE provides a comprehensive set of tools for coding, debugging, and testing your Java programs. Some popular Java IDEs include Eclipse, IntelliJ IDEA, and NetBeans. Choose the one that suits your needs and install it.
- Configure Your IDE: Once you have installed your preferred IDE, you may need to configure it to recognize the JDK you installed earlier. Refer to the documentation of your IDE for instructions on how to set up the JDK path.
Java Basics
To lay a strong foundation in Java, it is essential to grasp the basic concepts. In this section, we will cover some key topics:
Understanding Variables
In Java, variables are used to store data values. Before using a variable, you need to declare its type and name. Here's an example of declaring and initializing a variable of type int
:
javaCopy codeint
age
=
25;
Mastering Data Types
Java provides several built-in data types, including int
, double
, boolean
, and String
. Understanding and using the appropriate data types is crucial for efficient programming. For instance, you would use an int
to store whole numbers and a double
to store decimal numbers.
Control Flow
Control flow statements, such as if-else
, for
loops, and while
loops, allow you to control the flow of execution in your program. They enable you to make decisions and repeat code based on specific conditions. Here's an example of an if-else
statement:
javaCopy codeint
x
=
10; if (x > 0) { System.out.println("Positive number"); } else { System.out.println("Negative number or zero"); }
Exception Handling
Java provides a robust exception handling mechanism to handle runtime errors gracefully. By using try-catch
blocks, you can catch and handle exceptions, preventing your program from crashing. Here's an example:
javaCopy codetry { // Code that may throw an exception } catch (Exception e) { // Code to handle the exception }
Object-Oriented Programming in Java
Java's strength lies in its object-oriented nature. Understanding and applying object-oriented principles will help you write cleaner, modular, and maintainable code. Let's explore some key concepts:
Classes and Objects
In Java, everything is an object. A class is a blueprint that defines the structure and behavior of objects. An object is an instance of a class. To create an object, you use the new
keyword. Here's an example:
javaCopy codeclass
Car { String brand; public
void
startEngine() { System.out.println("Engine started."); } } Car
myCar
=
new
Car(); myCar.brand = "Toyota"; myCar.startEngine();
Inheritance
Inheritance allows you to create new classes based on existing classes, inheriting their attributes and methods. It promotes code reuse and hierarchical organization. Here's an example:
javaCopy codeclass
Animal { void
eat() { System.out.println("Eating..."); } } class
Dog
extends
Animal { void
bark() { System.out.println("Woof!"); } } Dog
myDog
=
new
Dog(); myDog.eat(); myDog.bark();
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables you to write more flexible and extensible code. Here's an example:
javaCopy codeclass
Shape { void
draw() { System.out.println("Drawing a shape..."); } } class
Circle
extends
Shape { @Override
void
draw() { System.out.println("Drawing a circle..."); } } class
Square
extends
Shape { @Override
void
draw() { System.out.println("Drawing a square..."); } } Shape
myShape
=
new
Circle(); myShape.draw(); Shape
anotherShape
=
new
Square(); anotherShape.draw();
Encapsulation
Encapsulation refers to the bundling of data and methods within a class, hiding the internal implementation details. It provides data protection and allows controlled access to the class members. Here's an example:
javaCopy codeclass
Person { private String name; private
int age; public String getName() { return name; } public
void
setName(String name) { this.name = name; } // Getter and setter for age } Person
person
=
new
Person(); person.setName("John"); System.out.println(person.getName());
Abstraction
Abstraction involves representing essential features without including the background details. It allows you to create abstract classes and interfaces that define common behavior without providing implementation details. Here's an example:
javaCopy codeabstract
class
Shape { abstract
void
draw(); } class
Circle
extends
Shape { @Override
void
draw() { System.out.println("Drawing a circle..."); } } Shape
myShape
=
new
Circle(); myShape.draw();
Java Collections
Java collections provide powerful data structures and algorithms to store, retrieve, and manipulate groups of objects efficiently. Let's explore some commonly used collections:
ArrayList
An ArrayList
is a dynamic array that can grow or shrink in size. It provides methods to add, remove, and access elements at specific positions. Here's an example:
javaCopy codeimport java.util.ArrayList; ArrayList<String> fruits = new
ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); System.out.println(fruits.get(0)); // Output: Apple
LinkedList
A LinkedList
is a doubly-linked list that allows efficient insertion and removal of elements at both ends. It is suitable for scenarios where frequent addition or removal of elements is required. Here's an example:
javaCopy codeimport java.util.LinkedList; LinkedList<String> countries = new
LinkedList<>(); countries.add("USA"); countries.add("India"); countries.add("China"); System.out.println(countries.getFirst()); // Output: USA
HashMap
A HashMap
is a key-value pair-based collection that provides constant-time performance for basic operations like get()
and put()
. It allows you to store and retrieve data using a unique key. Here's an example:
javaCopy codeimport java.util.HashMap; HashMap<String, Integer> population = new
HashMap<>(); population.put("USA", 331449281); population.put("India", 1393409038); population.put("China", 1444216107); System.out.println(population.get("India")); // Output: 1393409038
HashSet
A HashSet
is an unordered collection of unique elements. It does not allow duplicate values and provides constant-time performance for basic operations. Here's an example:
javaCopy codeimport java.util.HashSet; HashSet<String> cities = new
HashSet<>(); cities.add("London"); cities.add("Paris"); cities.add("Tokyo"); System.out.println(cities.contains("London")); // Output: true
Queue
A Queue
is a collection that follows the First-In-First-Out (FIFO) principle. It provides methods for adding elements at the end and removing elements from the front. Here's an example:
javaCopy codeimport java.util.LinkedList; import java.util.Queue; Queue<String> queue = new
LinkedList<>(); queue.add("John"); queue.add("Jane"); queue.add("Bob"); System.out.println(queue.peek()); // Output: John
Java File Handling
File handling allows you to read from and write to files on your computer. Java provides various classes and methods to perform file-related operations.
Reading and Writing Files
To read from a file, you can use the FileReader
and BufferedReader
classes. To write to a file, you can use the FileWriter
and BufferedWriter
classes. Here's an example of reading and writing files:
javaCopy codeimport java.io.BufferedReader; import java.io.BufferedWriter; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; public
class
FileExample { public
static
void
main(String[] args) { try { // Writing to a file
FileWriter
fileWriter
=
new
FileWriter("example.txt"); BufferedWriter
bufferedWriter
=
new
BufferedWriter(fileWriter); bufferedWriter.write("Hello, World!"); bufferedWriter.close(); // Reading from a file
FileReader
fileReader
=
new
FileReader("example.txt"); BufferedReader
bufferedReader
=
new
BufferedReader(fileReader); String line; while ((line = bufferedReader.readLine()) != null) { System.out.println(line); } bufferedReader.close(); } catch (IOException e) { e.printStackTrace(); } } }
Working with Directories
Java provides the File
class, which allows you to create, delete, and list directories. Here's an example:
javaCopy codeimport java.io.File; public
class
DirectoryExample { public
static
void
main(String[] args) { File
directory
=
new
File("my_directory"); if (directory.mkdir()) { System.out.println("Directory created successfully"); } else { System.out.println("Failed to create directory"); } File[] files = directory.listFiles(); if (files != null) { for (File file : files) { System.out.println(file.getName()); } } } }
Concurrency in Java
Concurrency is the ability to execute multiple tasks simultaneously. Java provides built-in features to support concurrent programming. Let's explore some of them:
Threads
A thread is a lightweight unit of execution within a process. Java allows you to create and manage threads using the Thread
class. Here's an example:
javaCopy codeclass
MyThread
extends
Thread { @Override
public
void
run() { System.out.println("Thread is running..."); } } public
class
ThreadExample { public
static
void
main(String[] args) { MyThread
myThread
=
new
MyThread(); myThread.start(); } }
Runnable Interface
The Runnable
interface is an alternative way to create threads in Java. It provides a single method run()
that defines the code to be executed by the thread. Here's an example:
javaCopy codeclass
MyRunnable
implements
Runnable { @Override
public
void
run() { System.out.println("Runnable is running..."); } } public
class
RunnableExample { public
static
void
main(String[] args) { Thread
thread
=
new
Thread(new
MyRunnable()); thread.start(); } }
Synchronized Keyword
The synchronized
keyword is used to control access to shared resources in a multi-threaded environment. It ensures that only one thread can execute a synchronized block of code at a time. Here's an example:
javaCopy codeclass
Counter { private
int
count
=
0; public
synchronized
void
increment() { count++; } } public
class
SynchronizationExample { public
static
void
main(String[] args) { Counter
counter
=
new
Counter(); Thread
thread1
=
new
Thread(() -> { for (int
i
=
0; i < 1000; i++) { counter.increment(); } }); Thread
thread2
=
new
Thread(() -> { for (int
i
=
0; i < 1000; i++) { counter.increment(); } }); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(counter.getCount()); } }
FAQs
1. How long does it take to learn Java programming language?
The time it takes to learn Java depends on various factors such as your prior programming experience, the time you can dedicate to learning, and the depth of knowledge you want to acquire. However, with consistent practice and dedication, you can become proficient in Java within a few months.
2. Is Java a difficult programming language to learn?
Java is considered to be relatively easier to learn compared to some other programming languages. It has a straightforward syntax and offers extensive documentation and resources. However, mastering Java requires time and effort, especially when diving into more advanced topics like concurrency and data structures.
3. What are the best resources to learn Java?
There are several excellent resources available to learn Java. Here are a few recommendations:
- Online tutorials: Websites like Oracle's Java Tutorials and W3Schools offer comprehensive Java tutorials for beginners.
- Books: "Head First Java" by Kathy Sierra and Bert Bates and "Effective Java" by Joshua Bloch are highly regarded books for learning Java.
- Online courses: Platforms like Udemy and Coursera offer a wide range of Java courses, both free and paid.
4. How can I practice Java programming?
To practice Java programming, it's important to write code regularly. Here are a few ways you can practice:
- Solve coding challenges on platforms like LeetCode and HackerRank.
- Work on small projects to apply your knowledge and gain practical experience.
- Join online coding communities or forums to collaborate with other Java developers and participate in coding discussions.
5. Can I learn Java without any prior programming experience?
Yes, you can learn Java even if you have no prior programming experience. Java is often recommended as a beginner-friendly programming language due to its readability and extensive documentation. Starting with the basics and gradually building your knowledge will help you grasp Java programming concepts effectively.
6. How can I stay updated with the latest Java developments?
To stay updated with the latest Java developments, you can:
- Follow Java-related blogs and websites, such as JavaWorld and Baeldung.
- Join Java developer communities on platforms like Stack Overflow and Reddit.
- Subscribe to newsletters and podcasts focused on Java programming.
Conclusion
Learning Java programming language requires dedication, practice, and a strong desire to expand your coding skills. By understanding the core concepts, exploring Java collections, mastering file handling, and delving into concurrency, you can become a proficient Java developer. Remember to make use of available resources, practice regularly, and embrace hands-on coding projects to solidify your knowledge. Happy coding!
Related Guides
How to Download Cracked Version of Tally ERP 9: A Comprehensive Guide
How to Write a Resignation Letter: A Comprehensive Guide
Data Science vs. Data Analytics: Unraveling the Key Differences
Discover the Top 10 Chat GPT Alternatives for Enhanced Conversations
How to Become a Pharmacist: A Comprehensive Guide
How to Become a Home Inspector: A Comprehensive Guide
How to Become a Border Patrol: A Comprehensive Guide